For more interesting questions, subscribe us by Signing Up.
1) Guess the output
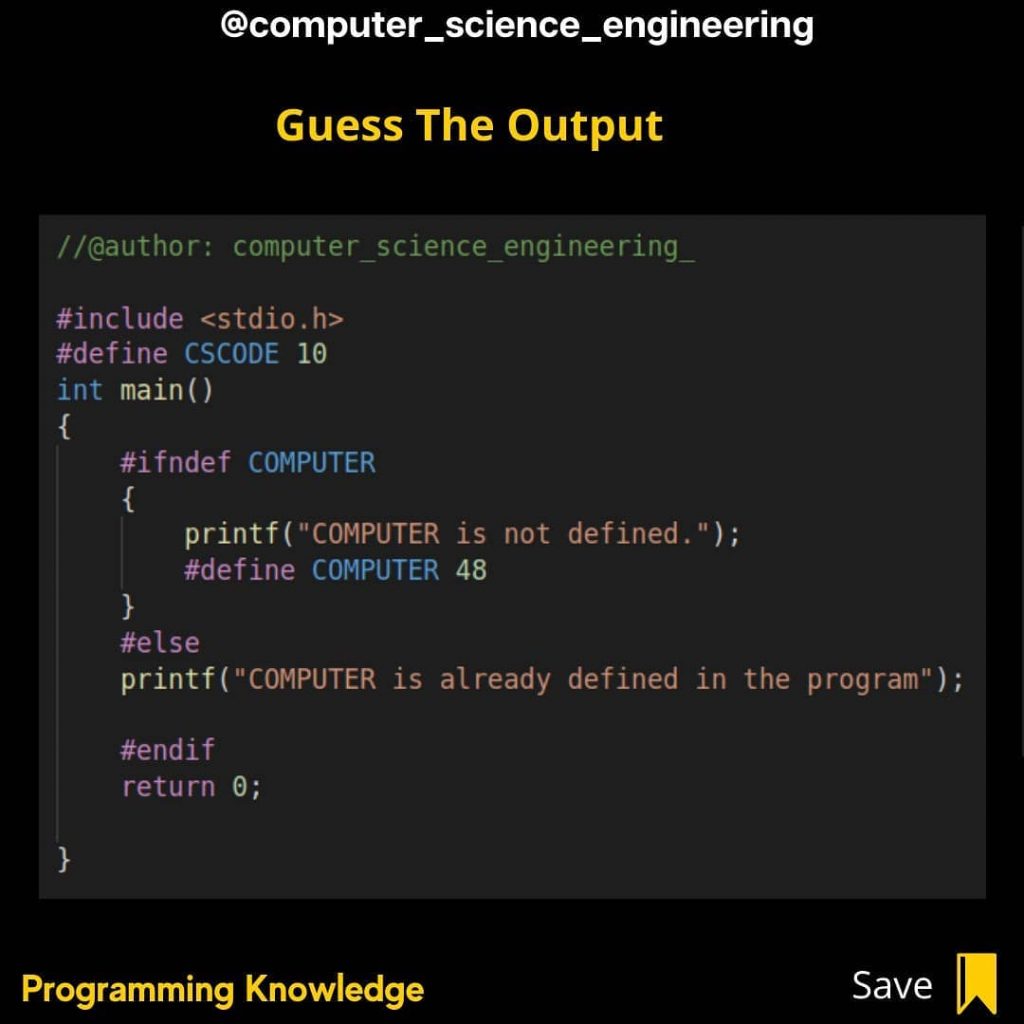
Before going to actual answer I would like to mention some key points about preprocessor directives.
We all know about Macros.
The C pre-processor is a macro pre-processor allows you to define macros that transforms your program before compilation.
#indef, #endif, #if, #else, #ifndef are the set of commands included or excluded in source code/program before compilation with respect to the condition.
If you want to check the expanded source code of above program on Linux, follow the following steps:
- gcc -E -o prefile.i your_code.c
- Open prefile.i file and go to the end of the file(first 800 lines are from stdio.h file or the files which are included in your source code/program).
- Check the content of main() function.
The word starts with # are processed before compilation part so don’t think like if-else statement, its like if-else statement to the preprocessor not compiler.
#ifndef COMPUTER means if COMPUTER is not defined then add this statements else add this statement to the source file.
So after the preprocessing stage, the source code will look like this and this expanded source code goes to the compilation step.
/* Note: above 800 lines are not included here
*/
int main()
{
printf("COMPUTER not defined.\n");
return 0;
}
So the output is COMPUTER not defined.
If you have any doubts, do let us know in the comment section.
2) Guess the output
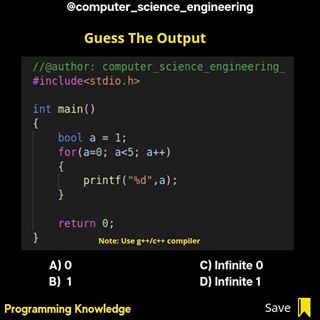
Click here to check full question and comments from our Instagram Page.
You might think that output of above code is 1 2 3 4 or 0.
But output of above code is option D i.e. Infinite times 1.
Why?
Before that run the following codes, so you will get some idea
#include<stdio.h>
int main()
{
bool a = 1;
a = a + 1;
printf("%d", a);
return 0;
}
Note: Try g++/c++ compiler to run above code or include <stdbool.h> header file for C Compiler.
The output of above code is 1.
Now replace a = a + 1 with a = a + 10, still you will get 1 output.
bool data type stores only true or false i.e. 0 or 1.
Any non-zero value will be treated as 1(true) and zero value will be treated as 0(false).
#include<stdio.h>
int main()
{
bool a = -10;
printf("%d", a);
return 0;
}
This code will give 1 as a output because -10 is non-zero number so it will treated as 1(true).
Now let’s come to the original problem.
#include<stdio.h>
int main()
{
bool a = 1;
for(a=0; a<5; a++)
{
printf("%d",a);
}
return 0;
}
Correct output of above code is 0 1 1 1 1 1 1……..infinite times.
Because a++ increases value of a=0 to a=1 and then for other all a++ value of a will always remains 1 and every time 1<5 condition becomes true.
3) Guess the output
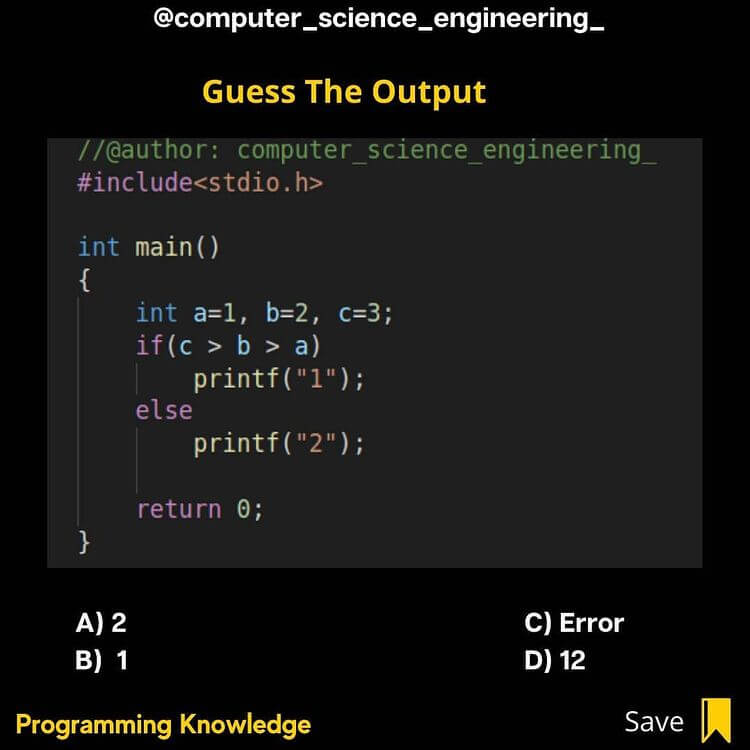
Output of above code is 2 not 1 or error.
It’s not like how we/humans think c>b>a i.e. 3>2>1 which is true but in programming this is different.
Let’s see how this expression (c>b>a) works unlike we think.
The associativity of ‘>’ is left to right i.e. above expression will be compiled like this
if( (c>b) > a)
c>b i.e. 3>2 is true which means 1(true).
Now the result of (c>b) i.e. 1 is compared with a.
if( 1 > a ) i.e. if( 1 > 1)
1>1 is false. Now if condition looks like
if(0)
printf("1");
else
printf("2");
So output of above code is 2.
For exercise run the following code
#include<stdio.h>
int main()
{
int a=1, b=2;
int temp1 = a > b;
int temp2 = b > a;
printf("%d %d", temp1, temp2);
return 0;
}
The output of above exercise is 0 1.
4) Guess the output
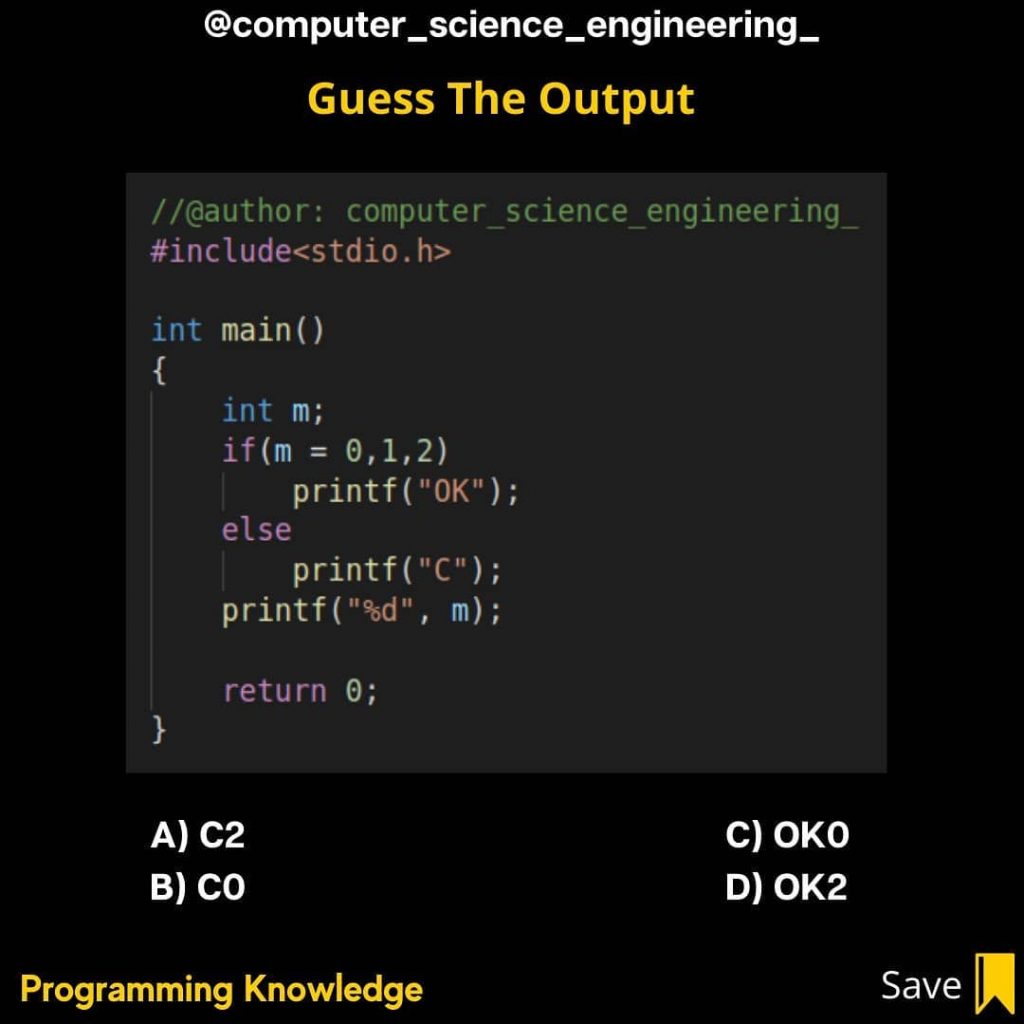
The output of above program is C) OK0.
Why?
At first zero will assign to ‘m’ then comma operator returns the last value which is 2 and condition becomes TRUE.
Try the following code
#include<stdio.h>
int main()
{
int m;
if(m=0)
printf("OK ");
else
printf("C ");
printf("%d", m);
}
//Output: C 0
For more programming questions and fun, follow us on Instagram. https://www.instagram.com/computer_science_engineering_/
More questions are coming soon…